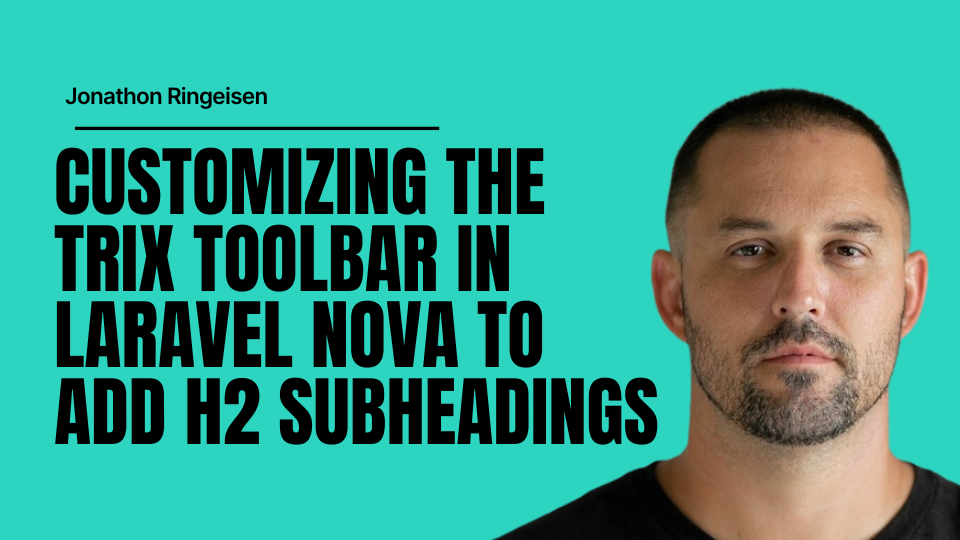
Customizing the Trix Toolbar in Laravel Nova to Add H2 Subheadings
If you've worked with Laravel Nova and wanted to customize the Trix editor toolbar, you may have realized that it's not as straightforward as you'd expect. By default, Trix doesn't allow adding custom buttons like H2 subheadings, but with a little tweaking, you can achieve it.
In this guide, I'll show you how to:
1. Add a custom H2 button to the Trix toolbar.
2. Ensure that the button applies a proper tag (instead of a div).
In this guide, I'll show you how to:
1. Add a custom H2 button to the Trix toolbar.
2. Ensure that the button applies a proper
tag (instead of a div).
3. Avoid common pitfalls like DOM errors and missing module imports.
Let's dive in!Step 1: Create a Custom Nova Tool
First, we'll create a custom tool to handle the toolbar customization.
Run the following command to create a new tool in your Laravel Nova app:php artisan nova:tool your-vendor/custom-prix-toolbar
Replace your-vendor with your namespace (e.g., wordsmith) and custom-trix-toolbar with the tool name.This will generate a new directory at:nova-components/CustomTrixToolbar
Step 2: Install Trix via NPM
Navigate to your tool's directory and install Trix as a dependency:cd nova-components/CustomTrixToolbar
npm install trix
Step 3: Update tools.js to Customize the Toolbar
Open the resources/js/tool.js file in your tool's directory and replace its content with the following:import Tool from './pages/Tool'
import 'trix'
// Boot the Inertia page if needed
Nova.booting((app, store) => {
Nova.inertia('CustomTrixToolbar', Tool)
})
// Register a custom block attribute for headings
Trix.config.blockAttributes.heading2 = {
tagName: 'h2'
}
// Add a custom H2 button to the Trix toolbar
document.addEventListener('trix-initialize', (event) => {
const toolbar = event.target.toolbarElement
if (!toolbar) return // Ensure the toolbar element exists
// Create a button for H2 subheadings
const buttonHTML = `
`
// Find the text tools group and insert the new button
const textTools = toolbar.querySelector('[data-trix-button-group="text-tools"]')
if (textTools) {
textTools.insertAdjacentHTML('beforeend', buttonHTML)
}
})
Step 4: Recompile Your Tool's Assets
After updating the tools.js file, recompile your assets to make sure the changes are applied.Run the following command:npm run dev
Step 5: Register the Tool in Your Nova App
To use the custom tool, you need to register it in your NovaServiceProvider.Open the app/Providers/NovaServiceProvider.php file and add the tool to the tools() method:public function tools()
{
return [
new \YourVendor\CustomTrixToolbar\CustomTrixToolbar,
];
}
Step 6: Test Your Custom Trix Toolbar
Now, navigate to your Nova app and open a resource that uses the Trix field.You should see a new H2 button in the toolbar. When you select text and click the button, it will apply an tag to the text.
Troubleshooting: Common Errors
Here are a few common errors you might encounter and how to fix them:
Error: Uncaught TypeError: Cannot read properties of null
Solution: Ensure you are accessing the toolbar after the Trix editor is initialized.
Error: Module not found: Error: Can't resolve 'trix'
Solution: Make sure you've installed Trix via NPM and imported it correctly.
Error: Call to a member function authorize() on string
Solution: Verify that your tool is correctly registered in NovaServiceProvider.Wrapping Up
Congratulations! You've successfully customized the Trix toolbar in Laravel Nova to include a custom H2 button. By following this guide, you can further extend the toolbar to include other buttons and customize the Trix editor to fit your needs.
If you found this guide helpful, feel free to share it with other developers!
Run the following command to create a new tool in your Laravel Nova app:
headings Trix.config.blockAttributes.heading2 = { tagName: 'h2' } // Add a custom H2 button to the Trix toolbar document.addEventListener('trix-initialize', (event) => { const toolbar = event.target.toolbarElement if (!toolbar) return // Ensure the toolbar element exists // Create a button for H2 subheadings const buttonHTML = ` ` // Find the text tools group and insert the new button const textTools = toolbar.querySelector('[data-trix-button-group="text-tools"]') if (textTools) { textTools.insertAdjacentHTML('beforeend', buttonHTML) } })
tag to the text.
Error: Uncaught TypeError: Cannot read properties of null
Solution: Ensure you are accessing the toolbar after the Trix editor is initialized.
Error: Module not found: Error: Can't resolve 'trix'
Solution: Make sure you've installed Trix via NPM and imported it correctly.
Error: Call to a member function authorize() on string
Solution: Verify that your tool is correctly registered in NovaServiceProvider.
If you found this guide helpful, feel free to share it with other developers!