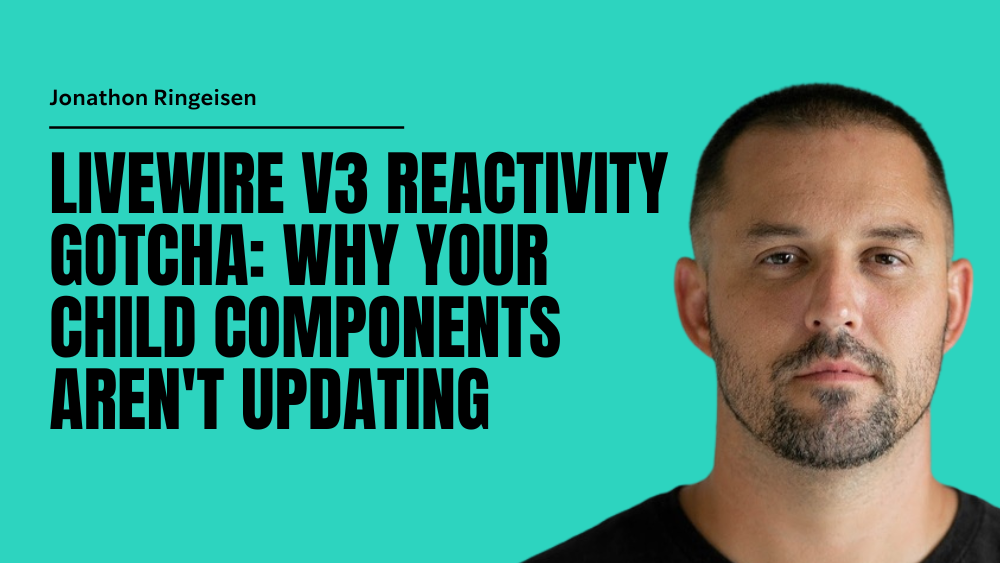
Livewire V3 Reactivity Gotcha: Why Your Child Components Aren't Updating
Livewire V3 has brought some exciting changes to the table, especially with its enhanced component-based architecture. As someone who loves building dynamic interfaces with Livewire, I was thrilled to dive into the new version. However, I recently ran into an issue that left me scratching my head for a while. I had a parent component that passed data to three child components using a computed posts method. Everything seemed fine until I updated the parent component and noticed that the child components weren't reflecting the changes. What was going on?
The Problem Encountered
The setup was straightforward: a parent component fetching a list of posts and passing them to child components for display. The posts were being calculated in a posts method, and I expected Livewire to magically handle the reactivity. But each time an update occurred on the parent component, the child components stubbornly displayed the old data. It felt like they were frozen in time.
At first, I thought I had messed up the data binding or made a typo. I checked everything—props, method names, event listeners—but nothing seemed out of place. Frustration was mounting, and I was beginning to wonder if I had misunderstood how Livewire's reactivity worked.
Digging into the Documentation
Determined to figure this out, I dove into the Livewire V3 documentation. That's when I stumbled upon a key detail: Livewire V3 is not reactive by default. Unlike previous versions, Livewire V3 requires you to explicitly mark properties as reactive using the #[Reactive] attribute.
This was a revelation. I had been assuming that Livewire would automatically track changes to computed properties and update child components accordingly. But Livewire V3 has taken a more deliberate approach to reactivity, giving developers more control over when and where to trigger updates.
Solution: Using the #[Reactive] Attribute
One important thing to note is that when passing collections as props, you might encounter this error:
Cannot mutate reactive prop
This is because Livewire V3 expects reactive props to be immutable. Since collections are inherently mutable, Livewire throws an error to prevent unintended side effects. The recommended solution is to convert the collection to an array before passing it to the child component. For example:
public function getPostsProperty() { return Post::latest()->get()->toArray(); }
This ensures the data remains immutable, and you won't run into the reactive prop mutation error.
The fix was surprisingly simple: I just needed to add the #[Reactive] attribute to the properties in my child components that were receiving the data. Here's what the updated child component code looked like:
class ChildComponent extends Component { #[Reactive] public array $posts; public function render() { return view('livewire.child-component'); } }
With the #[Reactive] attribute in place, the child components started updating as expected. Livewire was now tracking changes to the posts property and re-rendering the child components whenever the parent was updated.
Lessons Learned and Best Practices
This experience taught me an important lesson: don't assume reactivity. Livewire V3's decision to require explicit reactivity is a design choice that encourages better performance and more predictable behavior. But it also means that developers need to be mindful of where they apply the #[Reactive] attribute.
Here are a few takeaways:
- Use #[Reactive] on properties that depend on changing state or data.
- If child components aren't updating as expected, check if the parent properties are marked as reactive.
- Read the documentation! (Yes, I learned this the hard way.)
Conclusion
The move to explicit reactivity in Livewire V3 is a powerful feature that gives developers finer control over their components. But as with any new feature, there are nuances to learn. If you find yourself staring at stale child components, remember to check for the #[Reactive] attribute. Hopefully, this little gotcha helps other Livewire developers avoid the same confusion I faced.
Happy coding, and keep experimenting with Livewire V3!