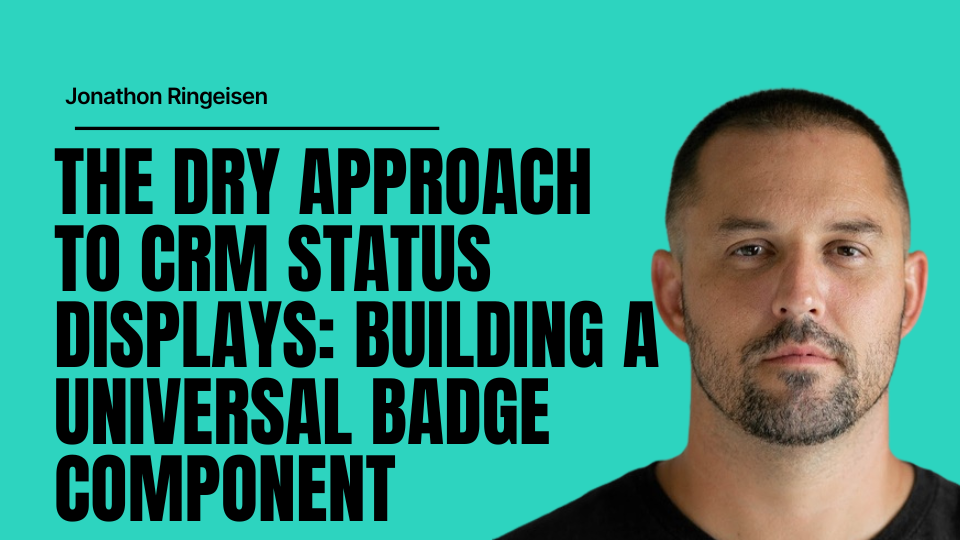
The DRY Approach to CRM Status Displays: Building a Universal Badge Component

Are you wrestling with a CRM that's bursting at the seams with multiple models, each flaunting its own set of statuses? If you've ever found yourself drowning in a sea of repetitive code updates just to change a simple status display, you're in the right place. This post is your lifeline to a more efficient, maintainable solution.
Imagine a world where you can update status displays across your entire application by tweaking just one piece of code. Sounds too good to be true? Well, buckle up, because we're about to make it a reality.
In this guide, we'll dive into:
- The power of Enums and dynamic types
- Creating a sleek, color-coded badge system for different statuses
- Implementing a centralized approach to status management
But before we leap into the solution, let's take a step back. I'll walk you through my original approach - warts and all. We'll dissect why it falls short, setting the stage for our journey towards code nirvana.
By the end of this post, you'll be armed with the knowledge to transform your CRM's status management from a sprawling mess into a lean, mean, efficiency machine. Ready to say goodbye to tedious, error-prone updates and hello to streamlined, maintainable code? Let's dive in!
//TaskStatusBadge.vue
{{ startCase(status) }}
The Hidden Pitfall of Hardcoded Components
At first glance, our current component seems to work flawlessly. It displays the correct badge with the right color for each status. So, what's the catch?
The issue lies not in its functionality, but in its lack of flexibility and reusability. Let's break down the problems:
- Limited Scope: This component is tailored for a specific set of statuses. What happens when we need to add a new status or change an existing one? We'd have to modify the component itself.
- Repetitive Code: With 5 different badge components for various models, we're violating the DRY (Don't Repeat Yourself) principle. This leads to maintenance headaches and increased chances of inconsistencies.
- Scalability Concerns: As our application grows, creating a new component for each model's statuses or types becomes unsustainable.
The Vision: A Universal Badge Component
What if we could have a single, flexible component that could handle any status or type across all our models? Imagine a component that:
- Accepts a :type prop to specify the current status
- Takes a :types prop to define all possible statuses and their corresponding styles
- Automatically renders the correct badge based on these props
With this approach, we could:
- Use the same component for all models, reducing code duplication
- Easily add or modify statuses without touching the component's internal logic
- Ensure consistency across our entire application
In the next section, we'll dive into refactoring our current implementation into this versatile component. Get ready to transform your codebase from a collection of rigid, single-use components into a flexible, maintainable system that can handle any status or type you throw at it!
The Power of Dynamic Badges: A Deep Dive
The Power of Dynamic Badges: A Deep Dive
Let's explore our newly refactored component and the supporting code that makes it truly dynamic and reusable.
1. The Universal Badge Component
// Badge.vue
{{ startCase(type) }}
This component is now flexible enough to handle any type of badge. It accepts two props:
- type: The current status or type
- types: An object mapping types to their corresponding color classes
2. Enum-Powered Type Management
// App\Enums\ClientTypes.php mapWithKeys(function ($case) { return [$case->value => ucwords(str_replace('_', ' ', $case->name))]; }) ->toArray(); } public static function badgeColors() { return [ self::LEAD->value => 'yellow-badge', self::PROSPECT->value => 'blue-badge', self::CUSTOMER->value => 'green-badge', self::INACTIVE->value => 'red-badge', self::REFERRED->value => 'purple-badge', self::VIP->value => 'indigo-badge' ]; } }
The ClientTypes enum serves two crucial purposes:
- It defines all possible client types, ensuring consistency across your application.
- The badgeColors() method maps each type to its corresponding color class.
3. Custom CSS for Badge Styling
/* Badge Colors */ .yellow-badge { @apply bg-yellow-50 text-yellow-800 ring-yellow-600/20; } .blue-badge { @apply bg-blue-50 text-blue-800 ring-blue-600/20; } /* ... other badge colors ... */
These custom classes leverage Tailwind's @apply directive to create reusable badge styles. This approach allows us to use dynamic classes that won't be purged by Tailwind's optimization process.
Putting It All Together
To use this system:
- Pass ClientTypes::badgeColors() from your backend to your frontend.
- In your Vue component, pass this data to the
component:
Conclusion: Embracing Flexibility and Maintainability
By refactoring our badge system, we've achieved several key improvements:
- Centralized Management: All badge colors are defined in one place (the enum), making updates a breeze.
- Reusability: Our
component can now be used for any model with statuses or types. - Scalability: Adding new types or changing existing ones no longer requires touching the component code.
- Consistency: With colors defined in the enum, we ensure consistent styling across the application.
This approach not only simplifies our current codebase but also sets us up for future growth. As your application evolves and new models or types are introduced, you can easily extend this system without accumulating technical debt.
Remember, the key to maintainable code often lies in creating flexible, reusable components and centralizing configuration. This badge system is just one example of how thinking ahead can save you countless hours of refactoring down the line.
So, the next time you find yourself creating multiple similar components, take a step back and consider: could this be solved with a more dynamic, centralized approach? Your future self (and your team) will thank you!