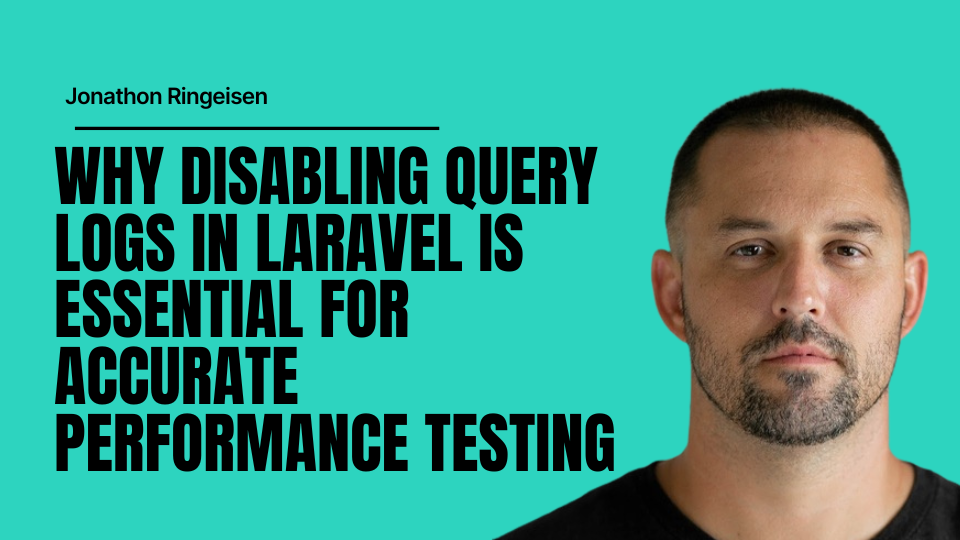
Why Disabling Query Logs in Laravel Is Essential for Accurate Performance Testing
When conducting performance tests in Laravel—especially memory usage tests—one of the most overlooked factors is the Laravel query log. By default, Laravel logs every database query that is executed, which can significantly skew performance metrics and lead to incorrect conclusions about the efficiency of your application. In this article, we’ll explore why disabling query logs is crucial during performance testing and how to do it properly.
What is Laravel's Query Log?
Laravel's query log is a feature that tracks and stores all executed SQL queries within your application. This is immensely helpful for debugging and optimizing database interactions during development.
However, the query log comes at a cost: each logged query consumes memory and increases processing overhead. While this is usually acceptable in a development environment, it becomes problematic when conducting memory usage or performance tests.
However, the query log comes at a cost: each logged query consumes memory and increases processing overhead. While this is usually acceptable in a development environment, it becomes problematic when conducting memory usage or performance tests.
How Query Logs Impact Performance Testing
- Increased Memory Consumption: The more queries your application executes, the more memory the query log consumes. This can lead to inflated memory usage metrics during testing.
- Skewed Performance Metrics: Performance tests aim to measure the efficiency of your code and database queries. Including the overhead from logging queries means you’re not getting an accurate picture of your application's true performance.
- Misleading Query Execution Times: The process of logging each query introduces additional processing time. This can make your queries appear slower than they actually are, leading to incorrect optimization efforts.
When Should You Disable Query Logs?
You should disable query logs whenever you are:
- Testing memory usage of queries or large database operations.
- Benchmarking the execution time of database queries.
- Running stress tests to evaluate application scalability.
How to Disable Query Logs in Laravel
Disabling query logs in Laravel is straightforward. By default, Laravel enables query logging through the database connection. You can manually disable it when necessary.
1. Temporary Disabling in a Controller or Command: To disable the query log within a specific section of your code, you can use the following method:
1. Temporary Disabling in a Controller or Command: To disable the query log within a specific section of your code, you can use the following method:
DB::connection()->disableQueryLog();
Here's an example in a controller:
use Illuminate\Support\Facades\DB; class PerformanceTestController extends Controller { public function testPerformance() { DB::connection()->disableQueryLog(); // Execute your queries here $results = DB::table('users')->get(); return response()->json(['data' => $results]); } }
2. Global Disabling for Testing Environment: If you want to ensure query logs are disabled throughout your entire test suite, you can modify the `AppServiceProvider`:
use Illuminate\Support\Facades\DB; use Illuminate\Support\ServiceProvider; class AppServiceProvider extends ServiceProvider { public function boot() { if (app()->environment('testing')) { DB::connection()->disableQueryLog(); } } }
3. Double-Check Query Log Status: After disabling the query log, it’s good practice to verify that it is indeed turned off:
if (DB::connection()->logging()) { throw new \Exception("Query logging is still enabled."); }
Additional Best Practices
- Use Database Transactions in Tests: When testing database interactions, use transactions to reset the database state between tests. This helps maintain clean test environments and avoids unintended side effects.
- Profile Queries with Database Tools: Instead of relying on Laravel’s query log, use database profiling tools such as MySQL EXPLAIN or third-party monitoring services for more accurate insights.
- Keep Debugging Tools Disabled: Ensure that debugging packages like Laravel Debugbar are disabled during performance testing to minimize their impact on the results.
Conclusion
Query logs are a powerful feature in Laravel, but they can distort performance test results by increasing memory consumption and skewing execution times. Disabling query logs during performance testing ensures that your benchmarks are accurate and that you are optimizing your application based on reliable data. By following the best practices outlined in this article, you can conduct more precise performance tests and make data-driven optimizations to your Laravel applications.